(Originally published as Stubbing For Fun, Profit, and Survival )
Anyone reading or watching the news these days know that IT project budgets will be tightening further than you may already be experiencing. While proponents of some languages and platforms claim to provide lower development costs, many others firmly believe that it is the habits of the developers who prefer those environments (more than the environment itself) that leads to rapid results. One of these habits is the proper use of method stubs. By proper, let’s define that as stubs that facilitate the development process first, and checking off “done” on a project plan second.
Stubbing Defined
Half the time someone asks me if I am the person who wrote a particular article it is a manager or analyst rather than a developer. Since the next person who asks you what you mean by “stubbing” may be using this article as their only reference, I thought I would add something that makes it easy to clarify this technique, and some fodder that will allow you to either argue or support my particular view on the topic…
Wikipedia defines a stub as “a piece of code used to stand in for some other programming functionality”. Sadly, the use of stubs in “general software development and testing” is thrown in as an after-thought. They give much more emphasis to its use in distributed computing, which is logical in the sense that the project failure rate is much higher if stubs aren’t used in distributed application development, and some techniques, such as SOAP-based web services require them as part of the API. In my mind, the only reason not to stub is when you can write the final code from end to end as a single developer in a single day. Any task more complex requires some type of stubbing, the specifics of which will vary between teams.
Benefits of Stubbing
The following are just a few of the advantages of developing methods stubs that return a meaningful value. I’ve left out some as obvious (despite the fact that I often say the obvious bears repeating in development) and others that are a bit inflammatory (having worked many long hours fixing the issues of not stubbing properly I find it hard to be objective).
The Ability to Demonstrate Progress on Demand
Many developers will only stub their methods if they are working in a large team. One value of stubbing methods even when working alone is that not only will the code compile, the code will run and return meaningful values. While this may appear as a minimal value to code quality and speed of development, it can be an enormous advantage to demonstrate to non-technical user (i.e., those that determine your budget) exactly how much progress you have made. While the code may be in the same stage of completion with or without meaningful return values, it is far more acceptable to clients and management to explain that the demonstration is not ready for production than to have to constantly excuse test cases that fail because of a null value.
Facilitating a Test First Development Approach
Test first development is a very useful Agile method. By writing test cases first that check for valid values and then implementing code that moves the test cases from “fail” to “pass”, you will know that your methods are written correctly at the earliest point possible in the development process. By running your test cases automatically at each build you can then pinpoint many bugs immediately after they are written rather once your code is integrated into the full project. Integrated code takes longer to debug for many reasons, one of which is that everyone assumes it is someone else’s code, thus costing the time of multiple developers to locate the issue rather than the one developer who wrote it.
Parallel Development
While it can be argued that it is possible to develop in parallel without providing stubs, it is rare that the eventual integration of layers developed in parallel goes smoothly if stubs with meaningful return values are not used to test the contracts between teams early and often.
Weekends Off
Ok, this one won’t apply to everyone, but I bring it up because during the holiday weekend I wrote this article I was also debugging integration issues for the very reason that the points covered in this article were not followed by experienced developers. I understand that it was not their intent to cause more work, which is why the lessons here are important for both the newbies and seasoned professionals.
Misconceptions about Stubbed Methods
Stubbing is Only Necessary at the Model Layer
As mentioned under Benefits of Stubbing, one advantage to stubs is the ability to demonstrate on demand. The HTML of a view object that is blank or displays “null” is far less likely to help your budget case than seeing “John Doe”, which is obviously not real data but is meaningful data.
When working in parallel development it may be tempting to point at a project plan that has dependencies being delivered in perfect synchronization as consider stubbing a controller with null because the model should be ready before the view is integrated. In the event that everyone does not hit their milestone exactly (gee, how often does that happen?) development can fall behind waiting for someone else to catch up. Alternatively, if you get ahead of your own schedule you will not be able to progress until your dependencies are at the same plan stage as you. If all developers stub their code with meaningful value at every step, they are not dependant on others until the actual point of integration, and then only in the event of bugs, which are far fewer if stubbing was occurring at each layer and each stage.
Null is Not a Stub
There are two phrases that will raise the blood pressure of many team leads and development managers. The first is “it works on my machine”, and the second is “it compiled for me, so I checked it into source control”. It isn’t the mere fact that a developer’s machine will not be the one used in production that is the issue here (though, it is one of the obvious things that still need to be pointed out often), it is that these phrases are only used when the code in question has broken the application as a whole. The most common cause of integration builds failing is a null value that should not be null. It may even (and probably will) compile within the integrated application. The failure point is when the application is run, a point that is far more costly and the a compilation failure because it can impact other developers (who have to trace their code to find the cause from someone else’s code), QA engineering teams (who have to reschedule testing because the application isn’t available), and likelihood of future team projects if this disaster occurs during a client demonstration.
The effort involved in adding and later removing:
//FIXME: Remove when data available if(value==null) {return “John Doe”;} else return value;
should total about 15 minutes, versus the average 1.5 man hours it takes to detect the issues caused after deployment. And 15 minutes is on the long side, as it took me 25 seconds to write the above example and should take no more than 1 minute to remove it.
“Foo” Is Only Missing an “L”
Our example above returns a meaningful value. Even in the case where full JavaDoc comments are omitted, any developer seeing “John Doe” returned has a pretty good idea how they can use the method. A return value of “foo” would give no such indication. Even if the method has full JavaDoc notations, the value “foo” is very unlikely to be of use to the client code. It may be helpful to consider that code examples that use “foo” generally use “bar” as the second value. “foo bar” is a homonym for FUBAR, which is an acronym for “F***** Up Beyond All Recovery”.
I recently worked on a project where stubs returned both null and “foo”. These values, coupled with generated JavaDoc comments rather than informative descriptions, led to delays in integration testing and the need to re-write 70% of the consumer code once integration began due to the assumed values not matching the actual values. A valid value returned by stubs for all methods would have prevented this and saved a substantial amount money (and one of my weekends).
Meaningful Stubs May End Up In Production
Some people believe that cleaning up stubbed code takes too much of their time, or that stubs can make their way into production by being missed. Looking at that second argument, it is only valid if comments are not used properly. All modern IDEs will provide a listing of comments that start with FIXME and TODO. Stubs that are commented with FIXME and cultivating the habit of reviewing these lists will always prevent stubs from making it to production and lead to cleaner code. Using these notations also allows for being able to quickly review code where stubs have been left and remove them when they are no longer needed.

Using a Stub Harness
If you don’t know how long it will be until you have data, consider writing a stub harness. A stub harness is a simple check to see if the class should use stubbed values before returning them. Whether to use the stubs or not can be set in a property file at a class or as a node in your deployment descriptor. This value can be set at a method level:
if(property.thisMethod.useStubs)
at the application level:
if(property.useStubs)
or both:
if(property.useStubs && property.thisMethod.useStubs)
The key to this approach is to make sure your build process will never allow these values to be set in either pre-production or production (or other applicable environments, depending on how your organization operates). Providing your processes include a regression tests in pre-prod, methods that are still stubbed will fail prior to going to production. It also allows you to switch off the stubs at any stage of development to run integration tests.
Conclusion
There are two inspirations for my article topics. Things that are cool, and things that are painful. In both cases my goal is to share the solution in the hopes of saving others the long, tedious discovery process that I and others have gone through. In the case of this particular topic, the tedious process isn’t the discovery but the realization that an approach that may seem at first glance to be time consuming is in fact time saving.

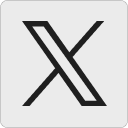



© Scott S. Nelson